C ++ PROGRAMMING
INTRODUCTION TO C++
➢ Introduction to the C++ programming language and its significance.
➢ Comparison with C and understanding object-oriented programming (OOP) concepts.
➢ Variables, data types, operators, and basic syntax in C++.
➢ Input and output operations using cin and cout.
CONTROL STRUCTURES AND FUNCTIONS
➢ Control Structures in C++:
➢ Conditional statements (if, if-else, switch-case)
and loops (while, do-while, for).
➢ Declaring, defining, and calling functions in C++.
➢ Overloading functions with different parameters.
OBJECT-ORIENTED PROGRAMMING (OOP) CONCEPTS
➢ Defining classes, creating objects, and accessing class members.
➢ Constructors, destructors, and member functions.
➢ Inheritance types (single, multiple, multilevel) and access specifiers.
➢ Polymorphism using function overriding and virtual functions.
POINTERS, REFERENCES, AND MEMORY MANAGEMENT
➢ Understanding pointers, pointer arithmetic, and references in C++.
➢ Pointers to objects, dynamic memory allocation, and deallocation.
➢ Memory management using new and delete operators.
➢ Introduction to smart pointers (unique_ptr, shared_ptr, weak_ptr).
OPERATOR OVERLOADING AND TEMPLATES
➢ Overloading operators for user-defined classes.
➢ Implementing operators like +, -, *, etc., for custom objects.
➢ Introduction to function templates and class templates.
➢ Overview of STL containers (vectors, lists, maps, etc.) and algorithms.
FILE HANDLING AND EXCEPTION HANDLING
➢ Reading from and writing to files using file streams.
➢ Handling file operations and error conditions.
➢ Understanding exceptions, try-catch blocks, and throwing exceptions.
➢ Handling exceptions to manage runtime errors.
ADVANCED TOPICS AND APPLICATIONS
➢ Introduction to multithreading concepts and thread synchronization.
➢ Creating and managing threads in C++.
➢ Principles like SOLID, design patterns, and best practices in OOP.
➢ Applying design principles in software development.
Projects and Application Development
➢ Emphasizing problem-solving and implementation of learned concepts.
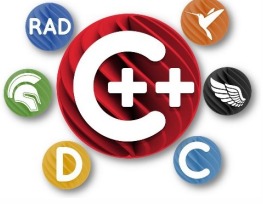

