INTRODUCTION TO IOS DEVELOPMENT
➢ Overview of iOS Development:
➢ Introduction to iOS, its significance, and the iOS development ecosystem.
➢ Understanding the iOS architecture and development tools (Xcode, Swift).
➢ Basics of Swift programming language: syntax, variables, data types.
➢ Swift features like optionals, functions, control flow, and error handling.
GETTING STARTED WITH IOS DEVELOPMENT
➢ Xcode IDE and Interface Builder:
➢ Understanding Xcode: IDE features, workspace setup, and navigation.
➢ Interface Builder for designing user interfaces (UI) using Storyboards.
➢ UIKit fundamentals: Views, ViewControllers, and their lifecycle.
➢ Implementing UI components (Labels, Buttons, TextFields) programmatically and via
Interface Builder.
WORKING WITH IOS COMPONENTS
➢ Implementing dynamic content using UITableView and UICollectionView.
➢ Customizing cells, handling selection, and managing data sources.
➢ Understanding navigation controllers, modal presentation, and segues.
➢ Passing data between view controllers and managing navigation flows.
DATA PERSISTENCE AND NETWORKING
➢ Working with Core Data for local data storage and retrieval.
➢ Managing user defaults, file handling, and data encryption.
➢ Making network requests using URLSession and handling JSON data.
➢ Integration with RESTful APIs and parsing JSON responses.
ADVANCED IOS DEVELOPMENT CONCEPTS
➢ Concurrency and Grand Central Dispatch (GCD):
➢ Managing asynchronous tasks, multithreading, and background tasks.
➢ Implementing GCD for efficient resource management.
➢ Implementing advanced UI components (StackViews, Collection View layouts).
➢ Animations using Core Animation, UIView animations, and transitions.
TESTING, DEBUGGING, AND DEPLOYMENT
➢ Unit testing with XCTest framework and debugging techniques.
➢ Instruments for performance profiling and debugging.
➢ App Deployment and App Store Submission:
➢ App provisioning, code signing, and preparing an app for submission.
➢ Understanding the App Store guidelines and submission process.
PROJECT WORK AND APP DEVELOPMENT
➢ Capstone Project:
➢ Developing a complete iOS application from concept to deployment.
➢ Applying learned concepts to create a functional app.
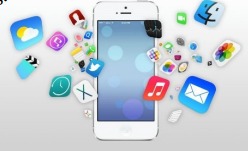

